Matrix AI Network integration tutorials — Part 1: Determine the addresses with activity and their respective balances for a specified number of blocks(Java + Maven), Intermediate level
by Delbypoc
This tutorial is for educational purposes only, I’m not responsible for any losses, damages, or other liabilities which may occur by using any info/code provided here(or anywhere else it is posted).
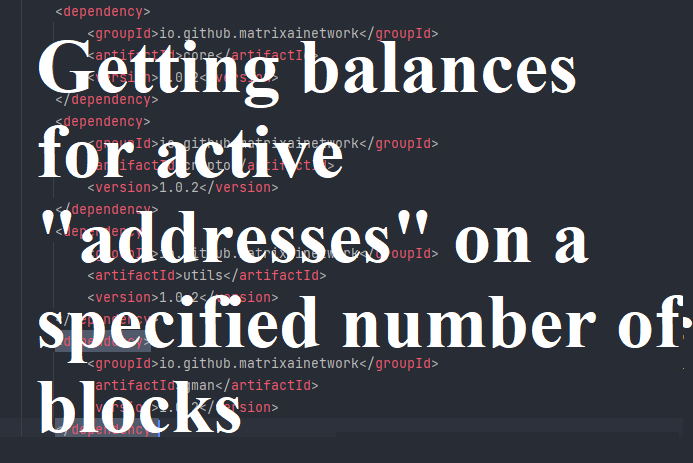
I’m under no circumstances a Java/Maven professional (or an Java/Maven Expert)!
The code is not production ready! (only for learning purposes)
In this guide, I’m going to show you how to determine the addresses who have activity (received MAN tokens) and their respective balances and display them in a sorted manner, based on maximum balance.
Before starting, I suggest taking a look at some maven fundamentals (https://maven.apache.org/ , we will use some of the libraries MATRIX AI NETWORK provided in their repositories).
You will also need an IDE that is compatible with MAVEN (or you will have to add the matrix ai network dependencies jars manually into the project, IntelliJ or Eclipse should work great)
Intellij: https://www.jetbrains.com/idea/
Eclipse: https://www.eclipse.org/
Let’s start by creating a new Maven project, you can skip this if you already know how to create a Maven supported project (/you can use any guide for creating a Maven compatible project, for example :https://www.jetbrains.com/help/idea/maven-support.html), some ways of doing it depending on your IDE would be:
For Eclipse: File-> New -> Project -> Maven Project -> Next -> check “Create an simple project box” -> write/choose any group Id/artifact Id -> in Package Explorer go into the src/main/java of the project and create an new class, I will name it GetTopHolders.java.
For IntelliJ: File -> New -> Project -> Java Project -> Create project from template -> Command Line App -> Pick a name -> Finish -> Right click on it in Project Explorer then Add Framework Support -> Select maven -> Ok -> Now you can open the generated Main.java file and rename it to GetTopHolders.java
We will now add the required dependencies in the pom.xml file (you can find more info about it on https://maven.apache.org/guides/introduction/introduction-to-the-pom.html, you can find those dependencies on maven repository https://mvnrepository.com/search?q=io.github.matrixainetwork)
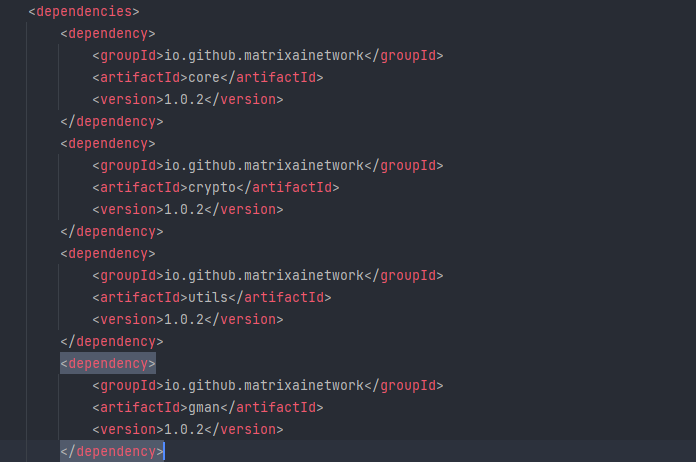
Let’s start coding:
I will initialize a provider first (I will pass in a public node of Matrix for use):

Then I will use the “provider” to get the current number of blocks and declare a parameter that sets the number of blocks to a number specified by us.
We will iterate over Transactions and verify which one has a Value higher than 0 so we can add the Addresses that received the amount into a HashMap so we can later query for balances:
(Will also print the current block we are looking into so we know that we didn’t get limited by the public node/stuck)
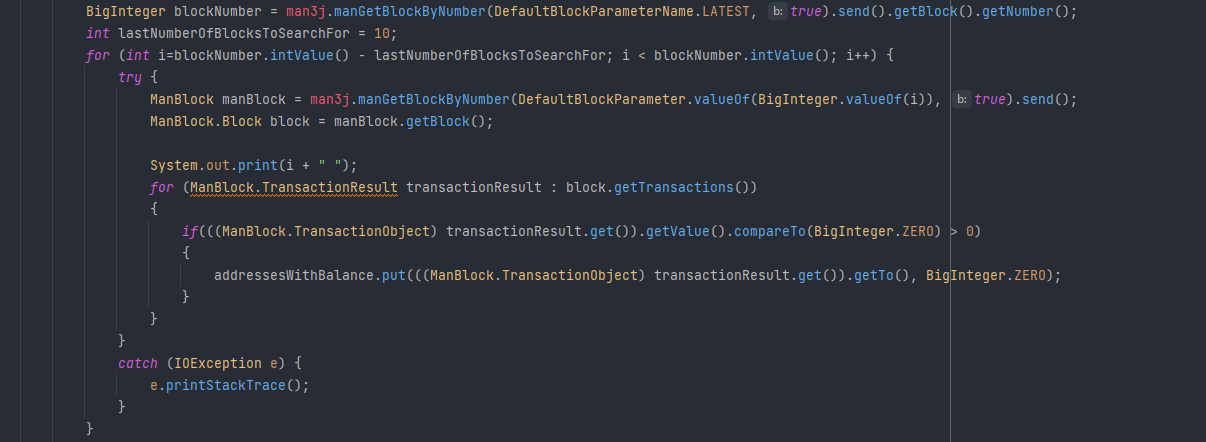
After we are done gathering addresses to query for balances (we know that those addresses got balances) we can run the getBalance method for each one of them then change the balance value accordingly :
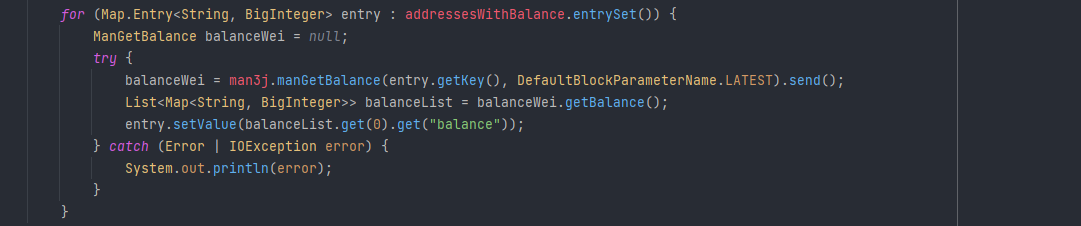
Now we can run a sorting (so the accounts get “sorted” based on their balance) and display them in the console:
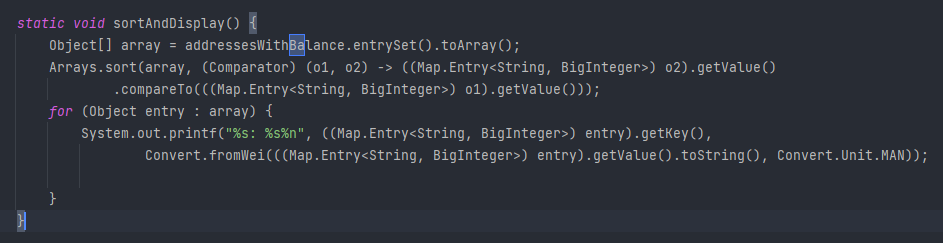
If everything goes well, we should see a result similar to this:
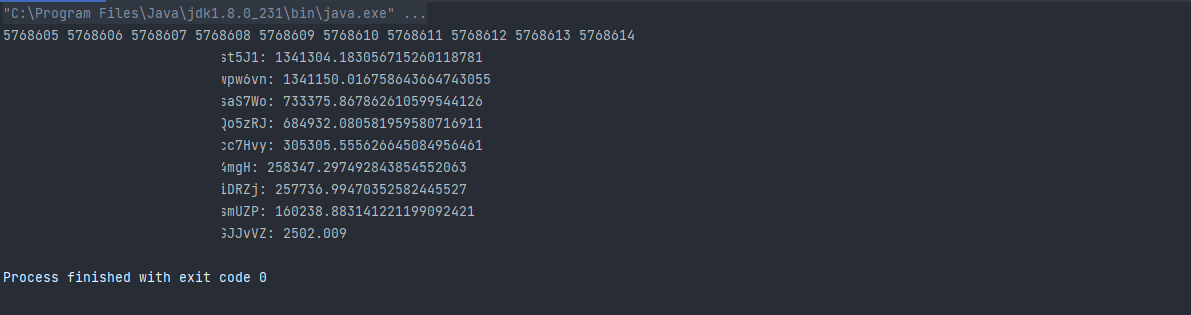
(blurred some of the addresses, in the photo it shows the addresses/balances for addresses that had activity in the blocks displayed in the first line, 10 blocks)
The single-threaded version is very slow so I also wrote a multi-threaded version, added a thread pool, changed the HashMap into a ConcurrentHashMap, and wrapped some of the code into a function named “searchForAddressesWithBalances”
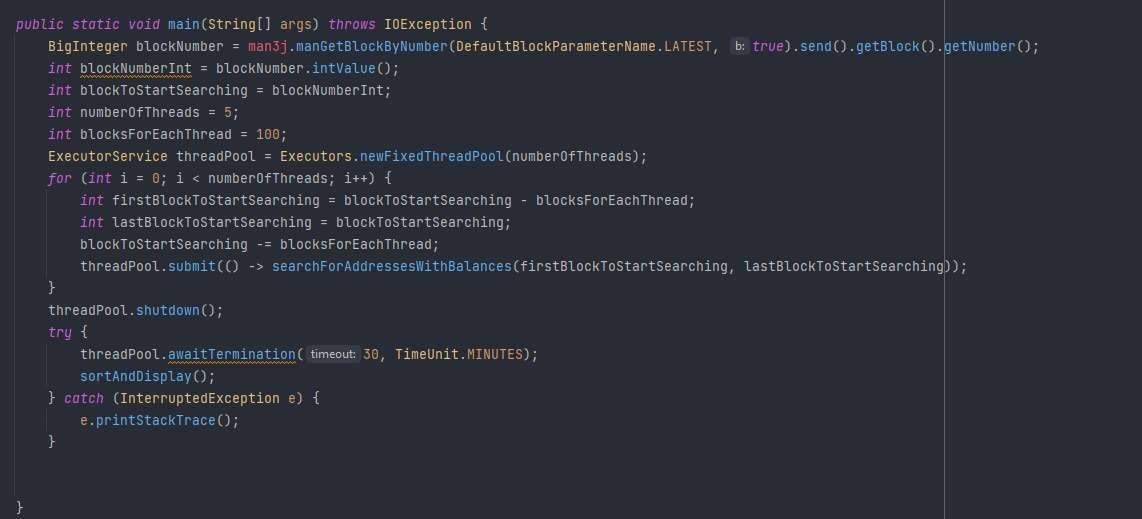
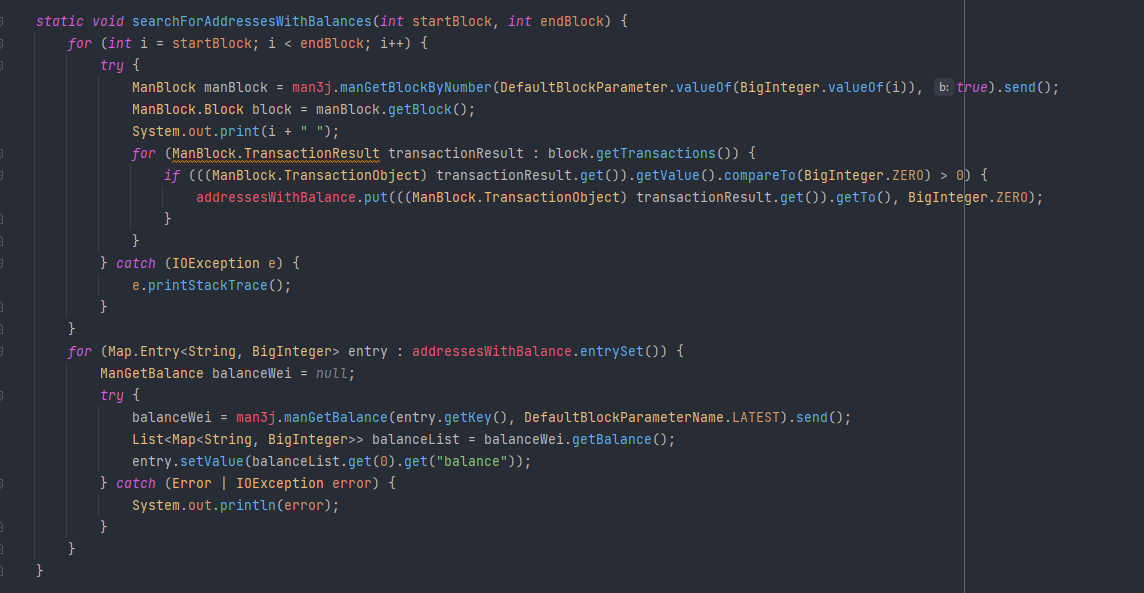
The multi-threaded version is also not that efficient as we will probably get banned/temporary limited from the “provider” node after many requests, an more efficient way of doing it would be storing the block information into a database (SQL/non-SQL) then querying for the addresses with the highest balances (doing it with your own node, not the public one).
Github code, single-threaded version :
Multi-threaded version:
Also, check out the official Matrix AI Network Developer Portal: